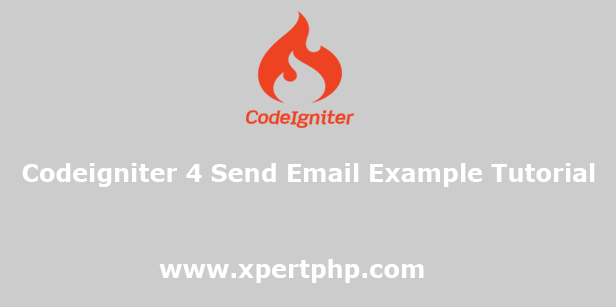
Codeigniter 4 Send Email Example Tutorial
In this tutorial, We will explain to you how to send email in CodeIgniter 4(Codeigniter 4 Send Email Example Tutorial). In this article, we are using the in-built email library of CodeIgniter 4.
Why Email is required, we are using it for sends new registered customers, forgot password, contact us, and invoices. Let us follow the below steps for send emails.
Overview
Step 1: Download Codeigniter
Step 2: Email Configurations
Step 3: Create Controller
Step 4: Create Routes
Step 5: Create Views Files
Step 6: Run The Application
Download Codeigniter
If you want to download or install CodeIgniter 4 then you can below Url.
How To Install Codeigniter 4 Using Manual, Composer, Git
Email configuration in Codeigniter
After the complete installation of Codeigniter 4. we have to mail configuration. now we will open the “app/Config/Email.php” file for Email Credentials for Gmail. See below changes in an Email.php file.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 | <?php namespace Config; use CodeIgniter\Config\BaseConfig; class Email extends BaseConfig { /** * @var string */ public $fromEmail; /** * @var string */ public $fromName; /** * @var string */ public $recipients; /** * The "user agent" * * @var string */ public $userAgent = 'CodeIgniter'; /** * The mail sending protocol: mail, sendmail, smtp * * @var string */ public $protocol = 'smtp'; /** * The server path to Sendmail. * * @var string */ public $mailPath = '/usr/sbin/sendmail'; /** * SMTP Server Address * * @var string */ public $SMTPHost = 'smtp.googlemail.com'; /** * SMTP Username * * @var string */ // Enter your email id from where you send email public $SMTPUser = 'Enter your email id'; /** * SMTP Password * * @var string */ // Enter your email's password public $SMTPPass = 'Enter your email's password'; /** * SMTP Port * * @var integer */ public $SMTPPort = 465; /** * SMTP Timeout (in seconds) * * @var integer */ public $SMTPTimeout = 60; /** * Enable persistent SMTP connections * * @var boolean */ public $SMTPKeepAlive = false; /** * SMTP Encryption. Either tls or ssl * * @var string */ public $SMTPCrypto = 'ssl'; /** * Enable word-wrap * * @var boolean */ public $wordWrap = true; /** * Character count to wrap at * * @var integer */ public $wrapChars = 76; /** * Type of mail, either 'text' or 'html' * * @var string */ public $mailType = 'html'; /** * Character set (utf-8, iso-8859-1, etc.) * * @var string */ public $charset = 'UTF-8'; /** * Whether to validate the email address * * @var boolean */ public $validate = false; /** * Email Priority. 1 = highest. 5 = lowest. 3 = normal * * @var integer */ public $priority = 3; /** * Newline character. (Use ā\r\nā to comply with RFC 822) * * @var string */ public $CRLF = "\r\n"; /** * Newline character. (Use ā\r\nā to comply with RFC 822) * * @var string */ public $newline = "\r\n"; /** * Enable BCC Batch Mode. * * @var boolean */ public $BCCBatchMode = false; /** * Number of emails in each BCC batch * * @var integer */ public $BCCBatchSize = 200; /** * Enable notify message from server * * @var boolean */ public $DSN = false; } ?> |
Create Controller
In this step, first, we will create a “Contact.php” controller after that we will create an index() and sendmail() method in this controller. When you call to index method that time we will return the view files. when you call the sendMail() that time we will pass the form data and send the mail.
app/Controllers/Contact.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 | <?php namespace App\Controllers; use App\Models\FormModel; use CodeIgniter\Controller; class Contact extends Controller { public function index() { return view('contact'); } function sendMail() { $to = $this->request->getVar('email'); $subject = $this->request->getVar('subject'); $message = $this->request->getVar('message'); $email = \Config\Services::email(); $email->setTo($to); $email->setFrom('xpertphp2018@gmail.com', 'Contact Us'); $email->setSubject($subject); $email->setMessage($message); if ($email->send()) { $msg = 'Email successfully sent'; } else { $data = $email->printDebugger(['headers']); //print_r($data); $msg ='Email send failed'; } return redirect()->to( base_url('contact') )->with('msg', $msg); } } |
Create Routes
Add the following route code in the āapp/config/Routes.phpā file.
1 | $routes->get('/contact', 'Contact::index'); |
Create Views Files
Finally, we will create a contact.php in the app/views directory. In this file, we will create the contact form using the bootstrap. for that you can see our example.
app/views/contact.php
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 | <!DOCTYPE html> <html lang="en"> <head> <title>Codeigniter 4 Send Email Example Tutorial - XpertPhp</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script> </head> <body> <div class="container"> <?php if (session('msg')) : ?> <div class="alert alert-info alert-dismissible"> <?= session('msg') ?> <button type="button" class="close" data-dismiss="alert"><span>Ć</span></button> </div> <?php endif ?> <form method="post" action="<?php echo base_url('contact/sendMail') ?>"> <div class="form-group"> <label>Your Email</label> <input type="text" name="email" class="form-control"> </div> <div class="form-group"> <label>Your Subject</label> <input type="text" name="subject" class="form-control"> </div> <div class="form-group"> <label>Message</label> <textarea rows="6" type="text" name="message" class="form-control"></textarea> </div> <button type="submit" class="btn btn-primary btn-block">Send</button> </form> </div> </body> </html> |
Run The Application
We can start the server and run this example using the below command.
1 | php spark serve |
Now we will run our example using the below Url in the browser.
1 | http://localhost:8080/contact |